JavaScript Bubble Sort Basics

Bubble Sort is a simple comparison algorithm used to sort through a given set of n elements in an Array. While simple to implement, bubble sort is not very efficient with a best case time complexity of a linear O(n) and worst case time complexity of O(n²) making bubble sort not ideal for larger lists. Bubble sort gets its name by the fact that as the algorithm is sorting the array the largest elements move or “bubble” to the right of the array.
If you are unfamiliar with the time complexity notation or better known as Big O notation, it is a mathematical measure of the execution of an algorithm with time and memory needed taken into account to solve a given problem size n.

The Algorithm
- Start with the first element of the array (index 0), compare the first element at index 0 with the next element of the array(index 1).
- If the current element is greater than the next element of the array, swap element values.
- If the current element is less than the next element, move to the next element.
Repeat step 1 until the entire array is sorted.


Implementation in JavaScript
1. Simple Implementation



2. Recursive Implementation


And now you’ll never forget bubble sort!
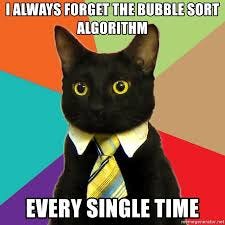
Resources: